Backend Development
Discover our expertly crafted MongoDB, Express and Node development course!
50+ Assignments & Practice Questions
3+ Projects
About the Course
Duration: 8 Weeks
Dedicated doubt solving sessions and support
This course also includes a comprehensive set of Questions related to interviews
Recorded + live lectures will be provided
Validity: Lifetime access
Comprehensive curriculum with different set of modules, keeping nitty gritty details in check.
Course Information
Experience hands-on learning as you build interactive user interfaces, design modular components, and deploy your projects to the web. This course empowers you with the expertise needed for a successful career in Frontend Development.
Learn the fundamentals of JavaScript, including variables, functions, ES6+ features, and event handling.
- JavaScript Overview
- Variables, data types, and operators
- JavaScript Functions
- let, var and const
- Hoisting and Scoping
- Closures
- Introduction to ES6+ features (let, const, arrow functions, template literals)
- Event Handling (click, hover, keydown, etc)
Explore advanced JavaScript topics such as working with APIs, asynchronous JavaScript, and object-oriented programming.
- Working with APIs (localStorage, timers, etc)
- Fetch API for making HTTP requests
- Error handling with try/catch
- Introduction to asynchronous JavaScript (callbacks, promises)
- Working with APIs and JSON
- Introduction to AJAX (Asynchronous JavaScript and XML)
- ES6+ advanced features (destructuring, spread/rest operators)
- Introduction to classes and object-oriented programming
- Modules and imports/exports
Introduction to Node.js, including asynchronous programming, environment setup, and running JavaScript files outside the browser.
- Introduction of NodeJS
- What is NodeJS and Why Use It?
- Difference between Synchronous and Asynchronous programming.
- How to run a Javascript file outside the browser.
- Difference between NPM and NPX?
- What are the inbuilt modules of NodeJs?
- Brief overview of event-driven, non-blocking I/O model of Node.js
- Environment Setup
Learn the basics of implementing I/O operations, creating servers, and error handling in Node.js.
- How to implement I/O operations synchronously.
- What is Blocking and Non-Blocking code in Node?
- How to make Node multi-threaded
- How to implement I/O operations asynchronously.
- How to Create a Server using Node.
- Error handling in Node.js
Explore routing, custom modules, and API design principles in Node.js.
- Implementation of Routing
- How to create your own custom modules in Node.
- Types of Dependencies.
- Using a Few Different Modules.
- API design principles and best practices
Learn about Express.js, including setting up a server, working with middleware, and using tools like Postman.
- Benefits of using Express instead of Node.
- What is Express?
- How to install & work with Postman and ThunderClient
- How to create a Server in Express.
- Express.js middleware and request-response lifecycle
Delve deeper into Express, learning about routing, CRUD operations, middlewares, and file structuring.
- Basic Routing
- How to handle CRUD Operations in Express.
- What are Middlewares in Express & Request Response LifeCycle?
- How to create our own Middlewares.
- File Structuring
- What are Environment Variables
Introduction to MongoDB, including installation, CRUD operations, and data modeling.
- What is MongoDB
- How to install MongoDB locally.
- Creating Local Database
- Implementation of CRUD operations.
- How to Create a Hosted DB using Atlas
- MongoDB data modeling and schema design
Learn how to connect MongoDB with Express using Mongoose, including schema design and validation.
- How to connect our database with Express.
- What is Mongoose?
- Creating a Simple Model
- Creating the documents and testing the model
- What is MVC Architecture?
- Mongoose schema, validation, and population
Explore error handling strategies and best practices in Express, including handling unhandled routes and middleware implementation.
- What is Error Handling
- How to Handle Unhandled Routes.
- Implementation of Error Handling using MiddleWares.
- Errors during development and Production
- Error handling strategies and best practices
Learn about authentication, authorization, and security best practices in Node.js and Express.
- Introduction to Authentication & Authorization
- How to Create Users and Manage Passwords
- Authentication using JWT
- Signing up Users and Logging up users.
- Advance postman Setup
- Authorization: User Roles and Permissions
- Password Reset Functionality: Reset Token
- Security best practices, such as input validation and sanitization
Learn how to send emails with NodeEmailer, manage user authentication, and implement email templating.
- Sending Emails with NodeEmailer
- How to update and Reset The password.
- How to delete the User
- Sending JWT via Cookies
- Email templating and email service providers
After completing the above modules, we will transition to working on industry-specific projects. These projects will extend the course duration by up to 2 additional weeks, making the learning experience more intriguing and effective.
Frequently Asked Questions
You can enroll in this course either by Live classes or you can visit us Offline to join the batch as we are offline too!
No, This course is completely designed to help you understand from basics to advanced level.
Please submit an inquiry or request a call back at a time that works for you if you are interested in any course or are unsure about your course choices. To help you find the course that is most appropriate for you, someone from Codehub Nexus will get in touch with you.
Access to the recordings is never taken away.
Please submit an inquiry or request a call back, our expert guide will connect with you to discuss more about the course & to help you choose what's right for you.
We accept all modes of payment including Credit/Debit card, UPI, NetBanking etc.
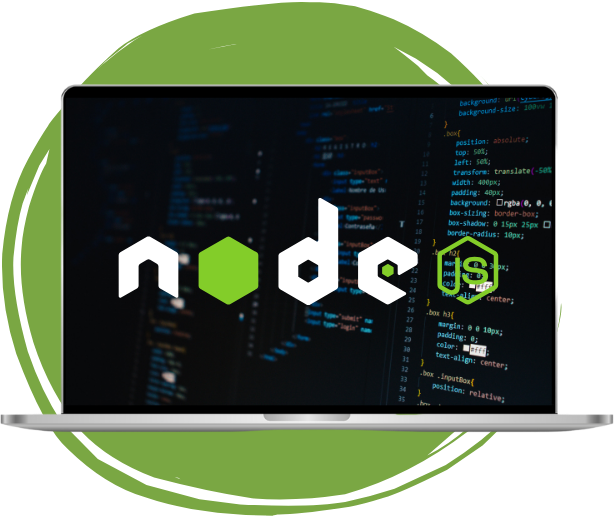